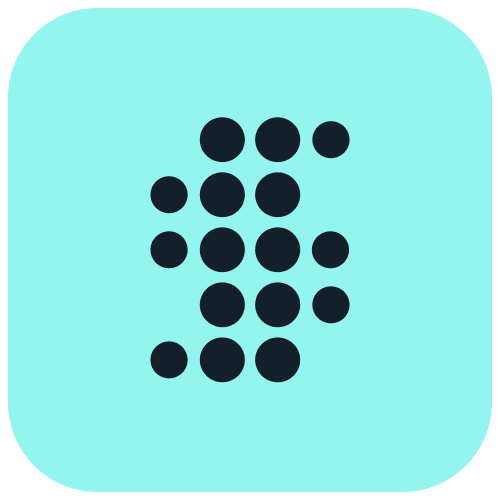
- Subscribe to RSS Feed
- Mark as New
- Mark as Read
- Bookmark
- Subscribe
- Printer Friendly Page
- Report Inappropriate Content
on
01-30-2025
07:46 AM
- edited on
01-30-2025
11:23 AM
by
jpacheco
Plugin – CustomMultiLevelFilter – Modify Filter Reset Behavior of Multi-Level Dashboard Filters
- Detect & Revert Lower-Level Resets: When a higher-level filter is updated, Sisense sets lower filters to “Include All.” The plugin prevents this and restores the previously selected members or exclude settings if it identifies a forced reset scenario.
- Timing Threshold: A configurable time window allows the plugin to classify filter changes that arrive quickly (and change only to “Include All”) as Sisense-forced resets rather than genuine user actions.
- Temporary Plugin Pause for “Reset Filters”: If the user explicitly clicks the Reset Filters button, the plugin stops reverting changes for a short window, ensuring that a intended full filter reset to the default state.
# Custom Multi-Level Filter Revert Plugin
## Description
This plugin detects and reverts Sisense’s automatic “forced resets” on multi-level filters to lower-level filters when a higher-level filter is changed, preserving only genuine, intentional user filter changes.
When a higher-level filter is switched from membership to another membership or to **Include All**, Sisense may automatically reset lower-level filters to **Include All**. This plugin reverts those lower-level filters back to their previous member or exclude state.
If the **Reset Filters** button is clicked, the plugin deactivates its revert logic for a short interval, allowing the reset to occur without plugin intervention.
A timing threshold is provided to accommodate delayed Sisense queries (JAQL calls) that might trigger a secondary filter-change event. If a second event arrives within that threshold and sets only member filters to **Include All**, it is treated as an automatic forced reset rather than a user-driven change.
## Installation
1. **Download the plugin:**
- Extract the compressed archive to `/opt/sisense/storage/plugins/`
- Or, in **Admin > System Management > File Management**, upload the extracted folder to the `plugins` directory.
2. **Wait for the plugin to load.**
3. **Refresh the dashboard.**
## Configuration
Two time-related variables, defined near the top of the main JavaScript file, influence this plugin’s behavior:
1. **autoResetThresholdMS** (initial default: 3000)
Sets how long after the first filter change event a second event is considered a forced reset. Any filters changed from membership to **Include All** within this period are reverted.
2. **resetButtonIgnoreMS** (initial default: 500)
Defines how long revert logic is ignored after the **Reset Filters** button is clicked. This ensures an intentional complete filter reset to the dashboard default filters is not reversed by the plugin.
These values can be adjusted to accommodate different JAQL response times and user interaction patterns.
If a dashboard has the dashboard object parameter `dashboard.disableFilterPlugin` set to true, the plugin will be disabled on this dashboard. This can be placed in the dashboard script.
## Limitations
- The plugin only affects multi-level filters. Single-level filters are not altered.
- The plugin specifically manages member-type multi-level filters and is not designed for other types of filters.
- Reloading the dashboard clears stored filter states, causing any accumulated data of filter states stored by this plugin to be lost for the new session.
- Adjusting `autoResetThresholdMs` involves a tradeoff. A higher value may risk treating rapid user actions as forced resets, while a lower value may allow some actual Sisense-forced resets to slip through.
- Sisense can issue multiple query results in quick succession for multiple levels, causing filters to be attempted to be set to **Include All** several times within the threshold. The plugin will detect and revert these resets repeatedly, which is normal for multiple asynchronous JAQL calls in sequence.
- The plugin does not block intentional user actions to set a filter to **Include All**. Only resets matching the time-based criteria are reverted.
- The code runs inside the prism.on("dashboardloaded", ...) event functions, ensuring it only applies once the Sisense dashboard is fully loaded.
- It sets up two timestamps—lastFilterChangeTimestamp and resetButtonClickedTimestamp—used to track recent filter changes and reset-button clicks.
- initialized: Captures the initial state (instanceid + levels) of every multi-level filter. This provides a baseline for comparison whenever filters are changed.
- filterschanged: The core logic:
- If the Reset Filters button was clicked recently (within resetButtonIgnoreMs ms), the plugin skips the revert logic (letting the user’s reset stand).
- Otherwise, the plugin checks how long since the last filter change:
- If the change occured within autoResetThresholdMs, and all changed levels went resetting from a “member” selection to “Include All,” the plugin classifies it as a forced reset and reverts to the old state.
- If exactly one filter level changed, it is assumed to be a genuine user action, so no filter change is performed.
- If multiple levels are changed at the same instant, only the earliest changed level is kept, and any subsequent levels changed to “Include All” are reverted.
- After reverting (or confirming) changes, the code updates a global window.oldFilterStates object so that the next filterschanged event knows the final “current” filter state.
- If the dashboard is reloaded, this state is not preserved, and the plugin starts over with loaded filter state. This is identical to standard Sisense filter behavior.
Usage of autoResetThresholdMs
- This threshold helps differentiate between genuine user actions and Sisense’s auto-resets. If Sisense triggers a forced reset a few seconds after the original user action (due to slow JAQL calls or large data sets), it still arrives within the threshold and gets reverted automatically.
Working with the Reset Button
- The user may want to revert all filters back to the set default filter state. Clicking the Reset Filters button triggers a short ignore window (resetButtonIgnoreMs). During this period, the plugin allows any Sisense-forced resets to persist, ensuring the user’s explicit intention of a full reset is honored.
Example Code Snippets
Detecting Multi-Level Filters
// Filters that have multiple levels (item.levels)
// are tracked by storing their instanceid and level states
if (item.levels && item.levels.length) {
window.oldFilterStates[i] = {
instanceid: item.instanceid,
levels: JSON.parse(JSON.stringify(item.levels))
};
}
Classifying Filter States
function getFilterType(filterObj) {
if (!filterObj) return "none";
if (filterObj.all) {
return "all";
}
if (
(filterObj.members && filterObj.members.length > 0) ||
(filterObj.exclude &&
filterObj.exclude.members &&
filterObj.exclude.members.length > 0)
) {
return "member";
}
return "none";
}
Reverting Forced Resets
The updateDashboard function is discussed in detail in this community article.
if (timeSinceLastChange < autoResetThresholdMs) {
// Check if all changed levels are member -> all
if (allAreMemberToAll) {
// Revert them
changedLevelIndices.forEach(function (lvl) {
newLevels[lvl].filter = JSON.parse(JSON.stringify(oldLevels[lvl].filter));
});
// Update Sisense
args.dashboard.$dashboard.updateDashboard(args.dashboard, ["filters"]);
args.dashboard.refresh();
}
}
Using This Plugin as a Basis for Your Own
This plugin combines custom JavaScript logic and Sisense’s plugin framework to alter default behavior. It can serve as a template for other Sisense plugins that need to:
- Listen for Sisense events such as initialized or filterschanged.
- Maintain custom state across events.
- Conditionally override or revert Sisense UI actions.
- Apply custom CSS or logic to the Sisense UI elements.
- With Sisense’s open plugin architecture, this approach can be adapted to create specialized behaviors for filters, widgets, or dashboards.
The CustomMultiLevelFilter plugin addresses a frequently requested feature: preserving lower-level filters when higher-level filters change. By leveraging Sisense’s event hooks and carefully distinguishing user actions from forced resets, it gives users greater control over multi-level filtering scenarios—especially in dashboards with deep hierarchies or many filter levels.